Wrinkle Solution with Marine Collagen
If you haven't met yet, let us introduce you to your new skincare best friend! The Wrinkle Solution with Marine Collagen is best known for its age-defying powers and ability to accelerate visible skin renewal, targeting expression areas and lines to recapture your youth. Used daily, the collagen-producing, salon grade actives and pure botanicals in this formula will ensure that you see and feel a noticeable difference in your skin.
These are some of its hero ingredients:
Aloe Vera
Aloe Vera is packed with antioxidants, anti-inflammatory properties and beneficial chemical compounds that make it a miracle for your skin. It intensely hydrates your skin by helping to retain and lock in moisture in the skin, as well as increases the water content of the outermost layer of skin. Hydrated skin is happy and healthy skin, meaning that sufficient moisture helps fight visible signs of ageing by keeping the skin firm, supple and more youthful.
White Tea
White tea contains a high amount of phenols and concentration of antioxidants which help strengthen collagen and elastin. White tea extract helps protect critical elements of the skin's immune system, helping protect your skin from oxidative stress. It helps protect and repair your skin from the inside out, making it one of the most effective agents in the anti-ageing market.
Marine Collagen
The word 'collagen' originates from the Greek word 'kolla' which translates to 'glue'. As the name suggests, collagen is the protein that keeps your skin strong and radiant, while repairing and protecting every layer of your skin. The marine collagen gives your skin structure and improves elasticity, helping to slow down and reverse the visible signs of ageing by stimulating the skin's natural collagen production.
If you haven't tried the Wrinkle Solution with Marine Collagen yet, do your skin a favour and experience the power of our age-defying moisturiser!
Featured Products
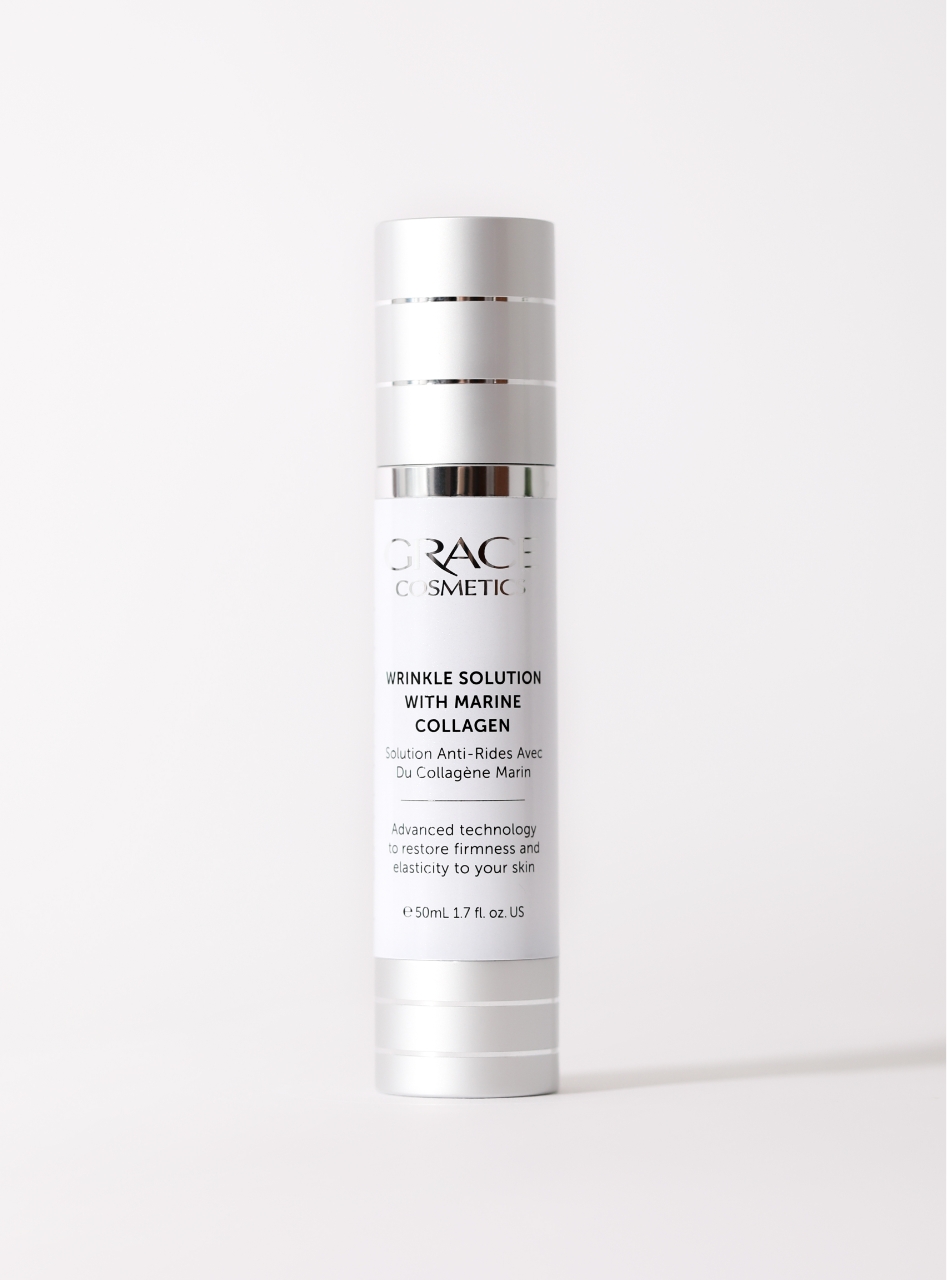